You will find many answers to the question where do I start to learn programming. I’ve been teaching programming for more than 20 years. So, here I’ll show you where do I think it is better to start according to my experience.
According to my experience and the feedback I get every year from my students, the answer is very simple: algorithms. You should start learning algorithms first, then you can focus on learning a programming language.
You will find several courses online, on different platforms, that teach you Python, or Java, or any other language. Those courses are not for beginners in programming (or coding). The problem you will have if you use that approach is this: you know a language, but when someone gives you a problem you don’t know how to apply that programming language to solve the problem.
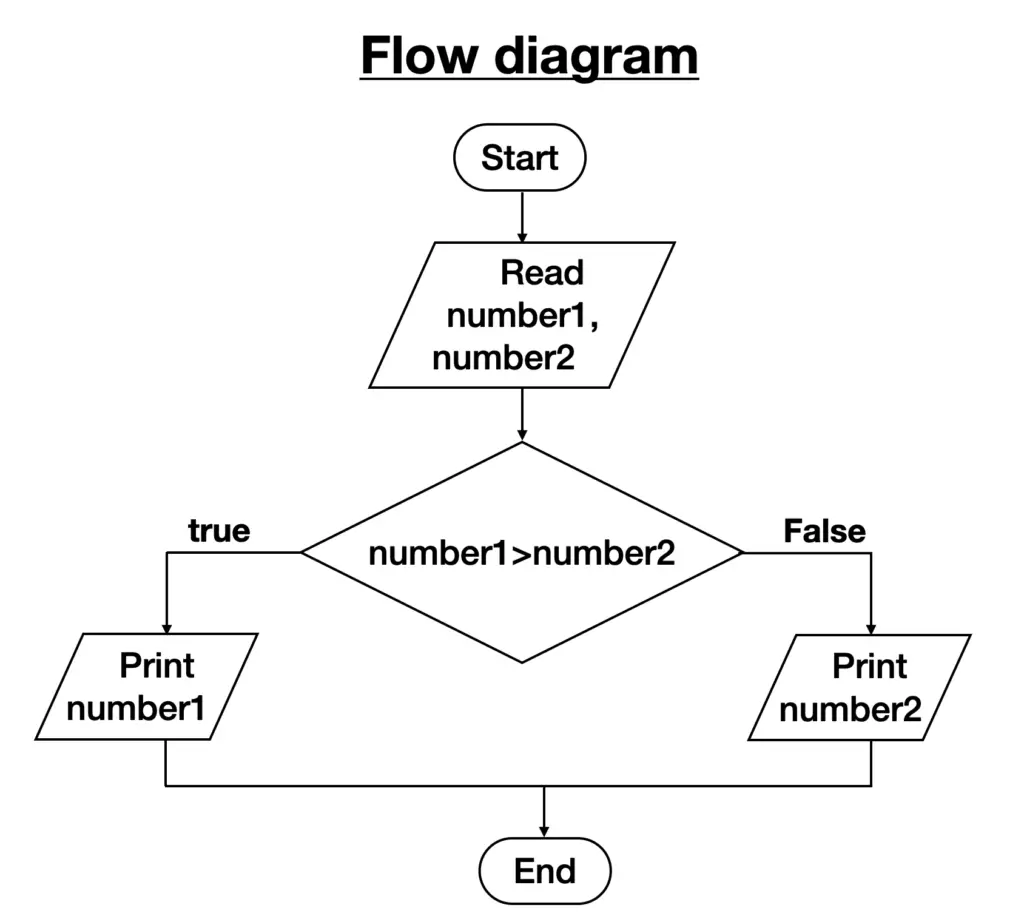
This will happen to you because the basis of programming is to solve problems, by using algorithms and a specific programming language (or languages). Notice that I wrote algorithms first, then the programming language, it is not a coincidence.
In programming, when you are given a problem to solve, you first must find out (design) the algorithm you are going to use to solve the problem.
Once you have the algorithm, and you are sure it will solve your problem, you decide what programming language you are going to use to implement the algorithm, and then solve the problem.
This approach you will find it mostly when you get formal education (school, university, etc.).
There are several people with programming knowledge, creating another type of materials. They usually focus on a specific programming language, or on showing you how to solve a specific problem. Most of that type of content is valuable. However, although it can help you, you will probably struggle when you try to solve a different problem. Because you will learn how to solve a specific problem instead of learning the fundamentals of programming. Learning the fundamentals will equip you with the tools you need to solve problems on your own, using algorithms and a programming language.
So, I’m putting together some lessons that will give you a holistic idea of what programming is, and the best way, according to my experience, to learn how to solve problems using programming languages (a.k.a. programming or coding).
Learn programming by learning algorithms first. Unit 1: Algorithms
In this unit, you will learn the basics of algorithms and how to use them to solve simple problems.
Also, you will see two ways of write algorithms: pseudo-code and flow diagrams.
Because this is an introductory unit, you should not get confused with the usual courses on algorithms and data structures. These are beginner-level lessons about algorithms. There is a lot more on algorithms than what is shown here. But the beginners must start at this level, to not be overwhelmed.
Unit 2: Applying algorithms
Once you know the basis of algorithms, it is time to learn how to apply them.
We apply algorithms to solve problems, by “teaching” a computer how to execute or follow the algorithm.
We “teach” a computer how to execute an algorithm, using a programming language.
A programming language is a tool we have available to “tell” the computer what to do. A computer only “understand” 0’s and 1’s. That is binary code. All the operations a computer carried out are specified in binary code.
Luckily, we don’t have to learn how to express algorithms in binary code.
We use, most of the time, a high-level programming language. This type of language has some similarities with the way we usually do certain things and have some similarities with mathematics.
When we have an algorithm written in a specific programming language, we use another program (called compiler or interpreter). A compiler will translate the code we wrote to a code that the computer can execute. An interpreter will read line by line the algorithm implemented in a programming language and execute it as it reads it.
In this unit, I’ll show you how to use the Java Programming Language to translate simple algorithms. You will also learn some of the basic concepts of Object-Oriented Programming.
Unit 3: Arrays
A program (an implementation of a certain algorithm or algorithms) have inputs and outputs.
The problem we must solve is: how to transform the input into the output?
For this problem-solving oriented approach, it is useful to have some mental models that we can use several times when trying to solve a problem.
Arrays are one of the ways we can store collections in a programming language. A collection will be several values (usually of the same type).
For instance, let’s say we have a collection of integer values. One example can be 1,2,3,4,5. The previous example is a collection of integers that contains exactly 5 integer values.
This type of data will help us to create some mental models that we can apply when we try to solve several problems. I called them basic algorithms.
The name “basic algorithms” is because we can use them as the basis for more complex algorithms that solve more complex problems.
Unit 4: Strings and Files
After learning basic concepts of Object-Oriented programming and basic algorithms, it is time to learn string and files.
String is a useful data type that we need to use to store a certain type of data.
Why do we need to learn this?
Remember that algorithms transform an input into an output. Inputs and outputs are data; therefore, we need to know how to represent different types of data in the programming language.
After that, we are going to study how to store data permanently. In this case, permanently means that even you switch off the computer, when you switch it on again, the data will be there, stored as a file in your computer.
Without the use of files (and databases -a special type of system used to store data-), we will have to enter the data every time we want to use it.
Imagine if you must enter 50 integers every time you want to execute your program. It will be a waste of time. That is why you can use files to store data that you will need to use repeatedly.
Conclusions on how to learn programming
The previous topics are my recommendation (in that specific order) for any person that wants to start getting into the awesome world of programming.
I’m using more than 20 years of experience, plus experience from my colleagues, plus the feedback I get all the time from my students.
I hope the lessons I prepared can be useful to you and can help you to start learning programming.
If you want to ask me anything, or just express your opinion, leave a comment below. I’ll make sure I get back to you.
H@appy learning!
Related content:
What is abstraction in programming and why is it important?