Collections is a very important topic in programming. Almost every code you will write will use collections in one way or another. In this article, you will learn what a collection is and how to use it in Java.
There is a general term widely used in the programmer’s community to refers to a collection: arrays. This happens because arrays are probably the most used type of collection. However, there are other types of collections, that is why I prefer to use the general term: collection.
So, what is a collection?
Collections
The definition that you can find in a dictionary is similar to this one: “A collection of things is a group of things.”.
All of us knows intuitively what a group of things is, let’s just say that are several things.
In programming, we are going to add to this group of things that they have the same type or at least related type.
For instance:
- a collection of numbers. All the “things” in this collection will be numbers.
- a collection of students. Every element in this group represents one student.
In programming, it won’t make sense to have a collection (group) with students, computers, and cars. If you must model and use the three different types of objects, then you should have three different collections, 1 collection for each type of object.
We can use collections to solve several problems in programming. Let’s first learn how to represent the collections in Java, and then we will solve one problem by using collections.
Unidimensional arrays in Java
The type of collection that is probably most used in programming is unidimensional arrays. Is the simplest type of collection and we can use it to create a more complex type of collection.
Let’s first see how data is stored in computer memory.

From the picture above, notice that the computer memory is represented sequentially. Each cell can store a value of a certain type and, we have access to the values through variables. If we print var1, we will get the value 5.
Imagine now that need the names of all the students enrolled in a class. If the students are three, we can have three variables (student1, student2 and student3). But, what about a situation where the class have 100 students?
Are we going to use 100 variables? Definitely not. For that case, we can use a collection of students.
Collection representation
Going back to the definition of collection, it is a group of objects or several objects of the same (or related) type.
So, we have a mechanism in programming languages, called arrays (in this unidimensional), to store several values of the same type.
See below the memory representation of such mechanism.

In this case, we will have a variable named “collection” (you can use any name for this variable). Using this variable, we will have access to all the elements of the collection by using an index.
If we want to access the first value of the collection, we can do so using collection[0]. To access the second one, you can use collection[1], and so on.
In most programming languages (but not all of them), the first element of the collection (array) is at the index 0.
Java syntax for collections
In Java, variables are defined by first declaring the type, and then the variable name. For instance, let’s declare an integer variable (or a variable with the type integer).
int var1;
For collections, the same applies. The only difference is that we have to tell the computer that is a collection by adding “[]” after the variable name.
int collection[];
After declaring de variable collection, we must initialize it before we can use it. We do so with the following line of code.
collection = new int[100];
In this case, we are “booking” space in memory for 100 integers. Using this variable, you cannot store more than 100 elements. Notice that if the index of the first element is 0, the last one is 99.
Once the collection is initialized, we can start using it.
collection[0] = 5; collection[1] = 8; System.out.print("The first element of the collection is "); System.out.println(collection[0]); System.out.print("The second element of the collection is "); System.out.println(collection[1]);
If we execute the code above in a console application, we will get the following result.
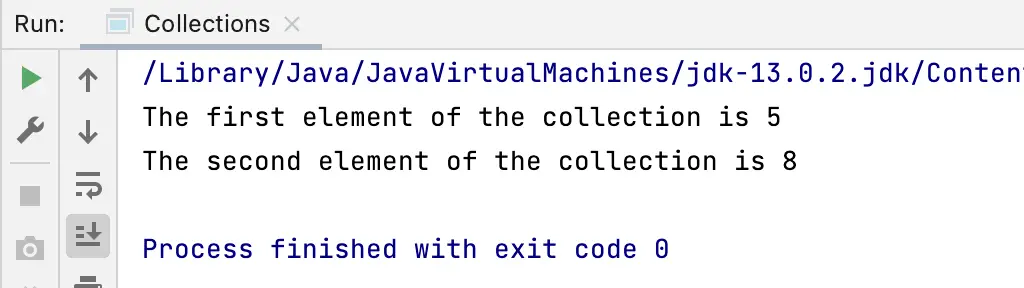
In the previous example, we use the indexes 0 and 1. It won’t make sense to use a greater index if the previous is not used. So, we always start by 0 and use the next index as necessary, until we get to the end (index 99 in the example).
Now let’s analyze the following problem. We have a collection of 100 elements, and we need to print all the elements.
Are we going to have 100 statements (or lines of code), one to print each element? No!
Just think about it, we previously learn a structure that allows us to repeat a block of code several times. Can we have a block of code that prints one element of the array, and then we repeat the execution of that line 100 times? Yes!
for (int index=0; index<100; index++){ System.out.println(collection[index]); }
If you remember, the control-loop variable is initialized, then the block of instructions is executed. After that, the increment is done (index++, same than index = index + 1), the condition (index<100) is checked and, if it is true, the block of instructions is executed again.
From the previous explanation, you must see that in every iteration the value of the variable index is incremented in 1, starting from 0. Also, the loop will stop when the condition (index<100) does not hold, which is when index equals 100. Therefore, the last value of index that will be used in the block of instructions (only 1 in this case) is 99.
Loops and collections (arrays) are used almost always together. So, to master the use of collections you need to master the use of loops.
Collections in the solution of a problem
Let’s solve the following problem.
Implement a console application that prints the average of 10 integer numbers using an array.
Let’s follow the steps from the last lesson of Unit 2:
- We first identify the classes,
- then design an algorithm for each operation.
- Only after that, we will use Java to translate our design
Because this is a simple example, we don’t need any to design any class. If you carefully read the problem, we just have to print the average of 10 numbers, so we decide that there is no class present in this problem.
Now, let’s design an algorithm.
To calculate the average of 10 numbers, we first must sum all the numbers, then divide by 10. One way to do it is to use the 10 indexes in only one line of code as follows:
collection[0]+collection[1]+ …+ collection[9]
But what if there are 1000 numbers in the collection? This approach is not scalable. This means you cannot use it when the input grows.
We don’t want to design algorithms that are not scalable. So, we have to use a different approach.
We know that loops can assist us in executing a block of instructions several times. What if we create a block of instructions that adds one number at a time in a “container”, and we repeat it as many times as numbers in the collection?
That can definitely be a solution to our problem. Let’s see the pseudo-code.
The solution
sum = 0 // this is our "container"
repeat, from i = 0 to number of elements in the collection
add element i to the container
The previous pseudo-code will sum all the elements, no matter how long the collection is.
After that, we can divide the sum by the number of elements, and we will obtain the average.
We initialize sum (the container) to 0 because 0 is the neutral for the sum operation. It means that when we add the first element of the array to the variable sum, it will be equal to that first element (sum = 0 + 2 = 2).
Our code in Java will be as follows:
public class Collections { public static void main(String[] args) { int collection[] = {2,3,4,5,6,1,8,2,9,9}; int sum = 0; for (int index=0; index< collection.length; index++){ sum = sum + collection[index]; } float average = (float) sum / collection.length; System.out.println("The average is: " + average); } }
Notice that the class Collections and the method main (public static void main) represents de console application, not the solution to calculate the average.
The line “int collection[] = {2,3,4,5,6,1,8,2,9,9};” initialize the collection with 10 integer numbers. Another way to do it, although not so clear is as follows:
int collection[] = new int[10]; collection[0] = 2; collection[1] = 3;
…
In the for-loop, we introduced the notation “collection.length“. This is a value that represents the number of elements of the array collection. So, if we assign 10 values to the array, “collection.length” equals 10, if we assign 500, then that variable (or attribute of the collection) will equal 500.
Let’s analyze the following line of code.
float average = (float) sum / collection.length;
What is the meaning of “(float) sum” before the division operator?
In Java, the division operator will return the same type of the operands. The variable sum was declared as an integer because the sum of integers is always an integer, the length of the array is also an integer, therefore the result of de division will be an integer (according to Java, LOL).
So, to get the real value of the division, we have to convert the value of the variable sum from an integer to a real value. That is the meaning of (float) sum. You can try removing (float) from the line of code and you will see how you get an integer as a result.
This algorithm that we just implemented here, is one of the five basic algorithms. I called them like that because most complex algorithms are created following a certain variation of these five. You can see the explanation and pseudo-code of the basic algorithms here.
It is important that you master these five basic algorithms before you go deeper into programming. It will ease the path for you.
Summary
In this lesson, we learned what a collection is, and how to implement unidimensional arrays (one type of collection) in Java.
We introduced one of the five basic algorithms. This one, and the other four, will be of immense use to you in your career as a programmer as you will use them most of the time.
If you have any questions or couldn’t execute the code I gave you here, leave a comment and I’ll reach to you to help getting started.
H@ppy coding!
If you like this short course, leave a comment below. It will help me to create better materials for you, and the ones who will come after.