A C# Console Menu Application is a great way to start learning programming. With a menu console application, you can easily test your code and see results after giving certain inputs.
In this post, I’ll show how to create a C# console menu application.
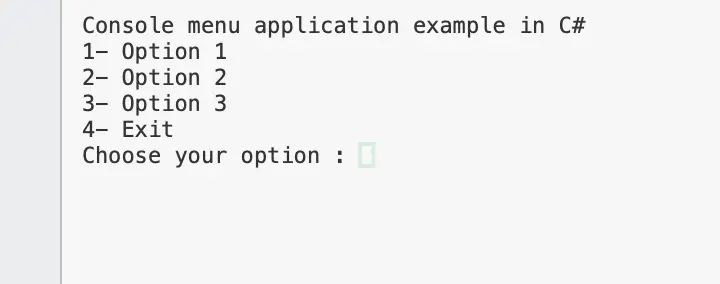
To create a menu for a C# console application, I recommend you the following steps:
- Print the menu options using an infinite loop
- get the option the user wants to execute from the keyboard
- use a switch-case statement to perform an action depending on the user input.
Table of Contents
- Printing the menu in the C# console application
- Add exception handling
- Implementing actions for each option of the console menu application
- Summary
Printing the menu in the C# console application
The first step for us to create a console app menu is to print the options on the screen.
We have several ways to print the options on the screen. My favorite way is to store the options in a string array. That will give us the flexibility to add or remove options as we need.
Each string will have the number of the option the user can choose, and a description of what is expected. For example: “1- Read information from file”. We will use this number later to know what action to execute.
Let’s see the code to print the options.
using System;
namespace menu
{
class MainClass
{
public static void printMenu(String[] options)
{
foreach (String option in options)
{
Console.WriteLine(option);
}
Console.Write("Choose your option : ");
}
public static void Main(string[] args)
{
Console.WriteLine("Console menu application example in C#");
String[] options = {"1- Option 1",
"2- Option 2",
"3- Option 3",
"4- Exit",
};
int option=0;
while (true)
{
printMenu(options);
}
}
}
}
In the code above we made three design decisions:
- Initialize an array of strings to store the options of the menu
- A method that prints all the options.
- An infinity loop that prints the menu when we execute the console app and ask the user to input an option.
The previous design model the way the menu console application works. A user enters an option using the keyboard, an action is executed according to the option and the user is asked for another option. If the option is to exit, then the computer finishes the execution of the console application.
Add exception handling
As we any code we write, we must make sure our menu console app is robust. Robust means in this context that our console application won’t crash, especially if there is a wrong user input.
For instance, if we are expecting an integer value, and the user input characters other than numbers, an exception will occur, and the program will crash. We need to avoid this type of behavior.
The way we create robust code is by using exception handling. See the code below.
using System;
namespace menu
{
class MainClass
{
public static void printMenu(String[] options)
{
foreach (String option in options)
{
Console.WriteLine(option);
}
Console.Write("Choose your option : ");
}
public static void Main(string[] args)
{
Console.WriteLine("Console menu application example in C#");
String[] options = {"1- Option 1",
"2- Option 2",
"3- Option 3",
"4- Exit",
};
int option=0;
while (true)
{
printMenu(options);
try
{
option = Convert.ToInt32(Console.ReadLine());
}
catch (System.FormatException)
{
Console.WriteLine("Please enter an integer value between 1 and " + options.Length);
continue;
}
catch (Exception)
{
Console.WriteLine("An unexpected error happened. Please try again");
continue;
}
}
}
}
Implementing actions for each option of the console menu application
After printing the menu, asking the user for an option, and handling possible errors, it is time to execute actions depending on the user input.
To do that, we are going to use a switch statement and implement one method for each option. In this way, our code will look better and will be easier to maintain. See the code below.
using System;
namespace menu
{
class MainClass
{
public static void printMenu(String[] options)
{
foreach (String option in options)
{
Console.WriteLine(option);
}
Console.Write("Choose your option : ");
}
public static void Main(string[] args)
{
Console.WriteLine("Console menu application example in C#");
String[] options = {"1- Option 1",
"2- Option 2",
"3- Option 3",
"4- Exit",
};
int option=0;
while (true)
{
printMenu(options);
try
{
option = Convert.ToInt32(Console.ReadLine());
}
catch (System.FormatException)
{
Console.WriteLine("Please enter an integer value between 1 and " + options.Length);
continue;
}
catch (Exception)
{
Console.WriteLine("An unexpected error happened. Please try again");
//Console.WriteLine(ex);
continue;
}
switch (option)
{
case 1:
option1();
break;
case 2:
option2();
break;
case 3:
option3();
break;
case 4:
Environment.Exit(0);
break;
default:
Console.WriteLine("Please enter an integer value between 1 and " + options.Length);
break;
}
}
}
private static void option3()
{
Console.WriteLine("Executing option 3");
}
private static void option2()
{
Console.WriteLine("Executing option 2");
}
private static void option1()
{
Console.WriteLine("Executing option 1");
}
}
}
See from the code above that there is one method per each option. Within this method, you can write the code you want to execute when the user chooses a specific option.
If you need to have more options, the steps are simple:
- Add the option to the string array options
- Add a new case statement within the switch for the new option
- Implement a method that executes the code for the new option
See an execution example in the picture below.
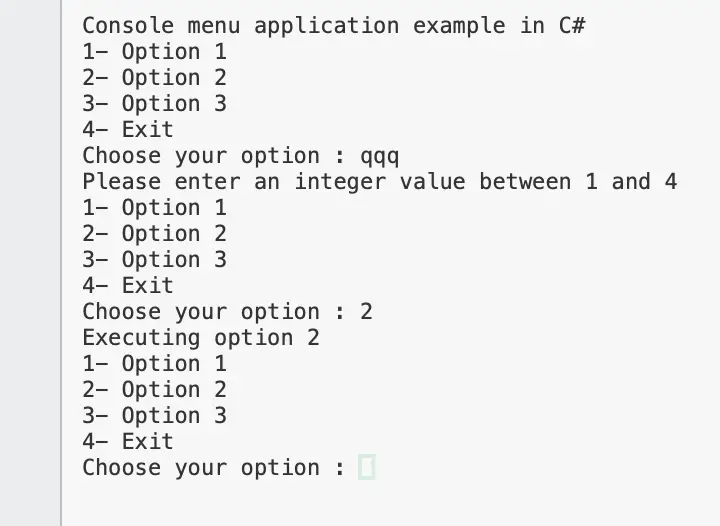
Summary
To create a C# Console Menu, follow the steps:
- Create a String array to store all the options available
- Create a method that prints the menu to the console using the string array from the previous step.
- Create an infinite loop to print the menu until the user chooses to exit the console application
- Use a switch statement to identify the option the user wants to execute
- Implement one method to execute the code for each available option
Related topics:
- Roadmap to learning programming: don’t learn a language
- The 5 basic algorithms in programming for beginners
- What is the best way to learn programming?
- Why use git in programming?
- OOP Concepts for beginners: Illustrated guide
- Where do I start to learn programming/coding?
- Object-oriented programming paradigm principles?