Arrays are very important in programming. Because of that, I’ll show you problems and solutions that require the use of arrays.
Arrays problem 1: Lenovo Company
Consider one of the examples of the previous lessons.
” Lenovo is a PC company that creates and sells laptops. The company has a record of all the laptops that the company creates with the following information: model, (screen) size, price. (…)” (complete example here) and the class laptop we designed in Java.
Let’s say the manager wants to know the following:
- The price of the cheapest laptop that the company is selling
- The average price of all the laptops
- How many laptops are cheaper than a given price?
Solution to the arrays problem
We are going to divide this solution into three parts. First the class Laptop, then the class Lenovo (that represents the company) and lastly, a console app to test the classes we implemented.
Class Laptop
First, we are going to implement the class Laptop. In the previous example, we already implemented it, so here I’m only going to show you again the code.
class Laptop{
private String model;
private int screenSize;
private float price;
Laptop(String model, int size, float price){
this.model = model;
this.screenSize = size;
this.price = price;
}
public float getPrice(){
return price;
}
}
Class Lenovo
Here we are going to discuss how to implement the class Lenovo. The main responsibility of this class is to hold/store all the information regarding the laptops that the company sells.
For this reason, we must have an attribute that will store several laptops. In other words, we need an array of laptops.
Remember that when we work with arrays (in Java), we must store the current number of objects that we added so far to the array. For that, we are going to use an integer variable (as a class attribute) named numberOfLaptops. This variable will have at all moments, the exact number of laptops that the company currently have.
Also notice, that in the constructor we have to initialize the array of laptops, and, in the beginning, there will be no laptops. Therefore, numberOfLaptops equals 0 when we create a new Lenovo object (in the constructor).
class Lenovo{
private Laptop laptops[];
private int numberOfLaptops;
public Lenovo(int maxLaptops){
laptops = new Laptop[maxLaptops];
numberOfLaptops = 0;
}
public void addLaptop(Laptop laptop){
laptops[numberOfLaptops] = laptop;
numberOfLaptops ++;
}
//The price of cheapest laptop that the company is selling
//Basic algorithm for summing
public float priceOfCheapestLaptop(){
float cheapestPrice = laptops[0].getPrice();
for (int i=1; i<numberOfLaptops; i++){
if (laptops[i].getPrice()<cheapestPrice){
cheapestPrice = laptops[i].getPrice();
}
}
return cheapestPrice;
}
// Average price of all the laptops
// Variation of basic algorithm for summing
public float averagePrice(){
float sum = 0;
for (int i=0; i<numberOfLaptops; i++){
sum += laptops[i].getPrice();
}
return sum/numberOfLaptops;
}
//How many laptops are cheaper than a given price?
// Basic algorithm for counting
public int howManyAreCheaperThan(float price){
int count = 0;
for (int i=0; i<numberOfLaptops; i++){
if (laptops[i].getPrice()<price) {
count += 1;
}
}
return count;
}
}
Notice the implementation of the three new methods. All of them are using what I called a basic algorithm.
This is one of the reasons why you should master the basic algorithms as soon as you can. I call them basic because they are used in many ways to solve problems.
Console application example to solve arrays problem
In this example, notice that we first must add laptops to the company (Lenovo) so we can then use the methods that we implemented to show the use of arrays to solve a specific problems.
public class Main {
public static void main(String[] args) {
Lenovo lenovo = new Lenovo(100);
Laptop laptop1 = new Laptop("M1", 13, 200);
Laptop laptop2 = new Laptop("M2", 15, 300);
Laptop laptop3 = new Laptop("M3", 15, 150);
lenovo.addLaptop(laptop1);
lenovo.addLaptop(laptop2);
lenovo.addLaptop(laptop3);
System.out.println("Price of the cheapest laptop: " + lenovo.priceOfCheapestLaptop());
System.out.println("Laptops average price: " + lenovo.averagePrice());
System.out.println("Laptops Cheaper than 300: " + lenovo.howManyAreCheaperThan(300));
}
}
Once you execute this sample console app, you will get the following as result.
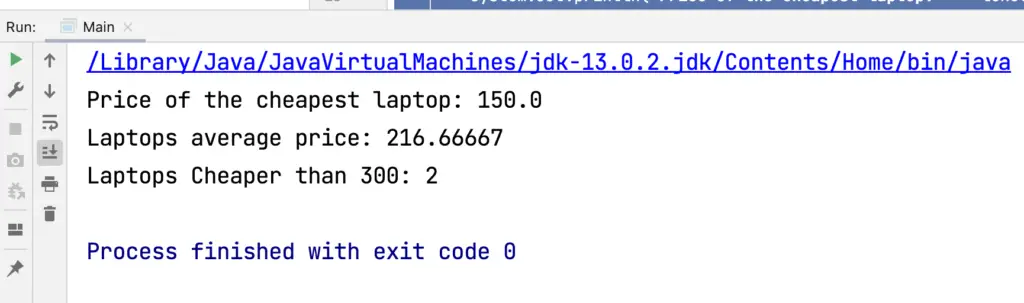
Arrays Problem 2: HR Department
The HR department of a certain company wants to provide employees with monthly payslips. The company knows for each employee the name, surname, employee number, basic salary and a collection of float values that states the allowances for the specific employee. In a meeting with the HR Director, the needs of the HR department as stated as follows:
- Create the payslip for a given employee. The payslip must be in the form: Full name, Employee number, Basic salary, Allowances, and tax. The tax can be calculated as 28% of the total income.
- Search for a specific employee.
- Calculate the average salary of the company.
As a guide to solve this type of problem, I recommend the reader to follow the following steps:
- Identify all the classes from the problem description and create a UML class diagram.
- Implement the classes using your favourite programming language.
- Create a console application that will use the implemented classes to test the classes implemented.
Solution
The solution is divided into four parts. First the UML diagram with all the classes, then we will use one section for each of three classes and lastly, a console app to test the classes we implemented.
UML diagram
UML diagrams are a way to describe classes. It is widely used and that is the reason why I want to introduce it to you at this point.
When you studied classes here, you learned that classes have three components: name, attributes and methods (or operations). You studied several examples in which I defined all three components to several classes.
UML is a standard used to represent classes. In this case, we are using a UML Class Diagram, representing each class and the relations between the class.
Relations between classes are of the scope of this article. For now, just use intuition to understand how the classes are related. A company has an HR department, and the department records the data of several employees. Those relations are represented in UML as shown in the picture below.
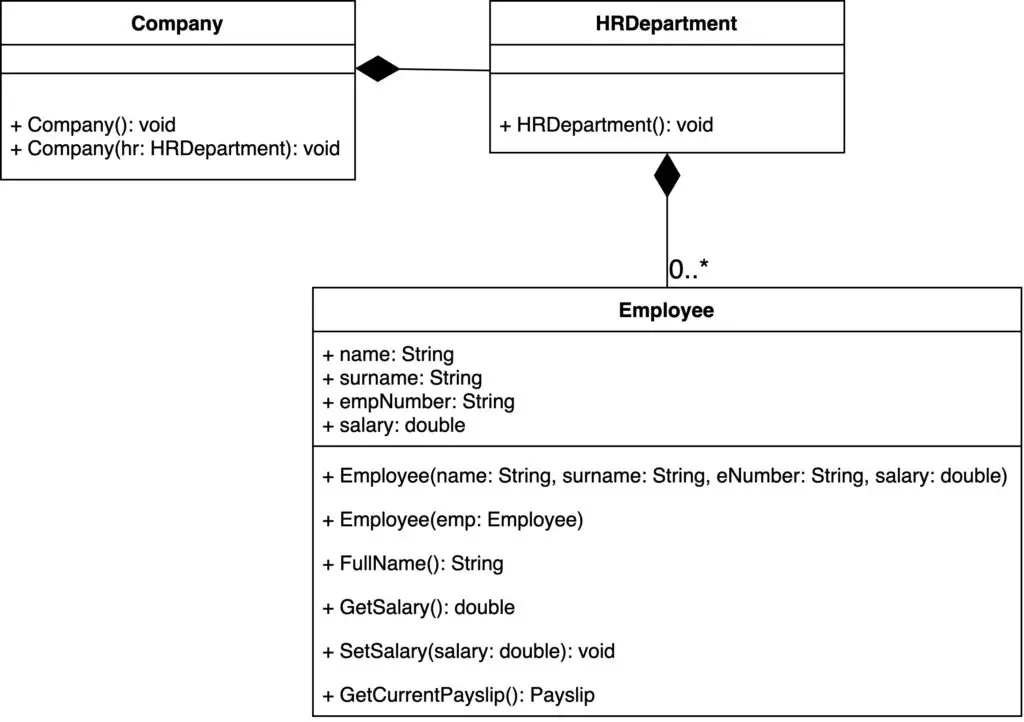
Once we have a class diagram, we can then translate that design into a specific programming language, Java in this case.
Class Company
As you can see in the code below, relations between classes are translated into class attributes.
The class company has an attribute that represents the HR Department.
The rest of the implementation of this class is straightforward and does not need the design of an algorithm to be completed. You just use the object hrDepartment, to invoke the respective operation.
public class Company { private HRDepartment hrDepartment; public Company(){ hrDepartment = new HRDepartment(); } public void addEmployee(Employee emp){ hrDepartment.addEmployee(emp); } public Employee getEmployee(String employeeNumber){ return hrDepartment.getEmployee(employeeNumber); } public String createPayslip(String employeeNumber){ return hrDepartment.createPayslip(employeeNumber); } public float averageSalary(){ return hrDepartment.averageSalary(); } }
Class HRDepartment
This class is the one that will implement what we call in programming the “business logic“. This is what implements the needs of the final user of the software we are creating.
To implement the business logic, we have to create algorithms that take an input and give the expected output, according to the business rules.
Let’s see one method: “getEmployee”. In this case, according to the business rules, an employee is uniquely identified by its employee number, so that is the input. The expected output is the employee (all the information related to the employee) if it exists.
Therefore, we have to create an algorithm to find out if the given employee number is an employee from the company.
Just think about this in this way, imagine you are the HR officer, you have a pile of files in front of you. Now you must search within each file (employees[i].getEmpNumber()) if the employee info in that specific file matches with the employee you are looking for (.equals(employeeNumber)). Because we are comparing strings, we have to use the method/operation equals instead of the comparison operator ==.
If we find one file that relates to the employee we are looking for, we give that file as a result (return employees[i];). If we don’t find any file, then we give no file as a result (return null;).
This algorithm is one of the five basic algorithms I suggest you learn. It is used in many situations.
Find below the whole implementation in Java of the class HRDepartment.
public class HRDepartment { private Employee employees[]; private int numberOfEmployees; public HRDepartment(){ employees = new Employee[100]; numberOfEmployees = 0; } public void addEmployee(Employee emp){ employees[numberOfEmployees++] = emp; } public Employee getEmployee(String employeeNumber){ for (int i=0; i<numberOfEmployees; i++){ if (employees[i].getEmpNumber().equals(employeeNumber)){ return employees[i]; } } return null; } public String createPayslip(String employeeNumber){ Employee emp = getEmployee(employeeNumber); if (emp!= null){ return emp.getPaySlip(); } else return ""; } public float averageSalary(){ float sum = 0; for (int i=0; i<numberOfEmployees; i++){ sum += employees[i].getBasicSalary(); } return sum/numberOfEmployees; } }
Class Employee
This type of class is usually called data class. Notice that the main responsibility of this class is to hold data related to the employee.
You mostly won’t find business logic in this type of class.
public class Employee { private String name; private String surname; private String empNumber; private float basicSalary; public Employee(String name, String surname, String empNumber, float salary) { this.name = name; this.surname = surname; this.empNumber = empNumber; this.basicSalary = salary; } public String getFullName() { return name + " " + surname; } public String getEmpNumber() { return empNumber; } public float getBasicSalary() { return basicSalary; } public String getPaySlip(){ return getFullName() + ", " + empNumber + ", " + basicSalary + ", " + basicSalary * 0.28; } }
Console application
Now is the time for us to test the class we just implemented.
To do that, we are going to use the following console application.
public class ConsoleApp { public static void main(String[] args) { Company company = new Company(); Employee emp1 = new Employee("John", "Doe", "00001", 350); Employee emp2 = new Employee("Jane", "Doe", "00002", 650); company.addEmployee(emp1); company.addEmployee(emp2); System.out.println("John Doe payslip"); System.out.println(company.createPayslip("00001")); System.out.println("Jane Doe payslip"); System.out.println(company.createPayslip("00002")); System.out.println("Is there an employee in the company with the employee number 00001?"); if (company.getEmployee("00001")!=null){ System.out.println("Yes, there is!"); } else { System.out.println("No, there is not an employee with that employee number!"); } System.out.println("Is there an employee in the company with the employee number 00003?"); if (company.getEmployee("00003")!=null){ System.out.println("Yes, there is!"); } else { System.out.println("No, there is not an employee with that employee number!"); } System.out.println("Averge salary of the company: " + company.averageSalary()); } }
The output after executing the previous console application will be as follows.
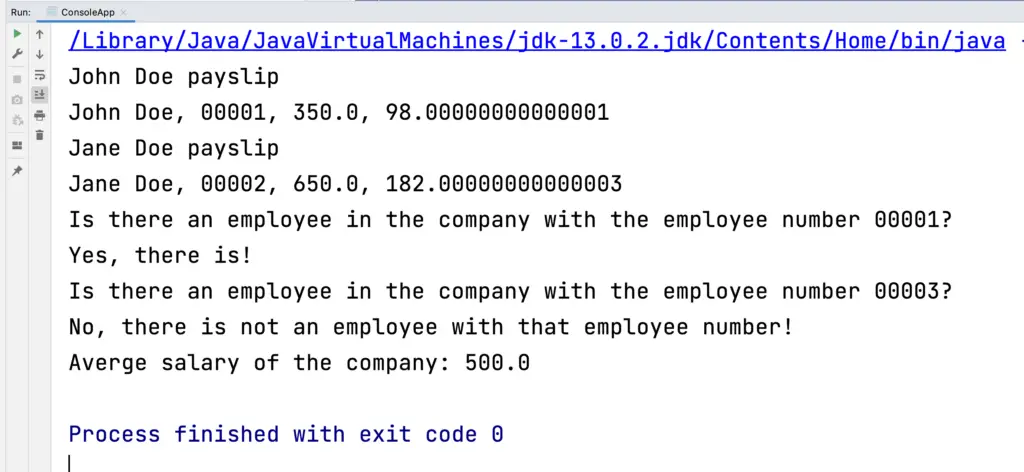
H@ppy coding!