Learn to create a menu for a python console application from scratch. Also, find explanations on each part of the code and some extra considerations.
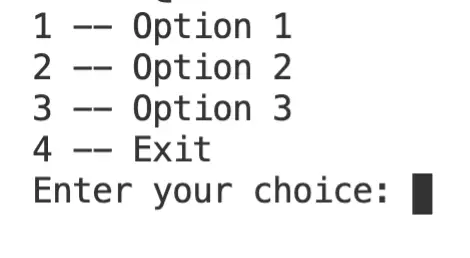
To create a menu for a python console application, I recommend you the following:
- Use an infinite loop to show the menu options.
- Ask the user for the options
- Use if-elif-else to determine what to do according to the user input.
It is a good practice to implement different functions to handle the menu options. The previous makes the code more readable and easy to understand.
The infinite loop
With the infinite loop, we are going to print the menu. Then, we ask the user to choose an option.
while(True):
print_menu()
option = int(input('Enter your choice: '))
Notice that we are using the function ‘print_menu’ to print the menu.
Why? The answer is simple: it makes the code clearer and easier to understand.
In the next section, we are going to implement the function ‘print_menu’.
Show the menu for our python application
We have several choices to show the menu options. I prefer to use dictionaries because it makes the implementation looks better. But you can do it in your favourite way.
First, I will define a dictionary with the options.
menu_options = {
1: 'Option 1',
2: 'Option 2',
3: 'Option 3',
4: 'Exit',
}
Now we can print the dictionary to show the user the available options.
def print_menu():
for key in menu_options.keys():
print (key, '--', menu_options[key] )
Did you see how nice looks the code above?
For me, it is a clean and clear code.
Another way you can implement this function is by using print statements. See below the example so you can compare wich implementation you prefer.
def print_menu1():
print ('1 -- Option 1' )
print ('2 -- Option 2' )
print ('3 -- Option 3' )
print ('4 -- Exit' )
Both ways work fine. You can use the one you prefer.
Determine what to do according to the user input
After the user enters his/her choice, we have to take action. Check below the sample code on how to do that with a simple if-elif-else code.
option = int(input('Enter your choice: '))
if option == 1:
print('Handle option \'Option 1\'')
elif option == 2:
print('Handle option \'Option 2\'')
elif option == 3:
print('Handle option \'Option 3\'')
elif option == 4:
print('Thanks message before exiting')
exit()
else:
print('Invalid option. Please enter a number between 1 and 4.')
Make your code readable and clear
Usually, we cannot handle a specific option from the menu in one line. Commonly, we have to write several lines of code to handle each option.
Because of that, our code can easily become unreadable.
An easy way to avoid that is to create a function to handle each option. In that way, our main code to handle user input will keep clear while to base-code grows.
See below how it will look like.
def option1():
print('Handle option \'Option 1\'')
def option2():
print('Handle option \'Option 2\'')
def option3():
print('Handle option \'Option 3\'')
…
option = int(input('Enter your choice: '))
#Check what choice was entered and act accordingly
if option == 1:
option1()
elif option == 2:
option2()
elif option == 3:
option3()
elif option == 4:
print('Thanks message before exiting')
exit()
else:
print('Invalid option. Please enter a number between 1 and 4.')
As you can see, it does not matter how many steps you will need to handle a specific option. The way you manage user input remains the same.
Extra considerations to create robust python applications
It is always good to create robust code. By robust, we mean that it is fault-tolerant.
In other words, it does not matter what the user input is; the program will never crash.
In our current implementation, the program will crash if we enter a letter instead of a number (option = int(input(‘Enter your choice: ‘))).
How can we avoid that?
The solution is easy, we just have to use exception handling.
try:
option = int(input('Enter your choice: '))
except:
print('Wrong input. Please enter a number ...')
If we use the code above, when the user enters something different than a number, a message will be printed requiring the user to enter a number.
Now we can say that our code is robust and, the application won’t crash.
The complete code for our menu for a python console application
Below is our final code for creating a menu for a python console application.
menu_options = {
1: 'Option 1',
2: 'Option 2',
3: 'Option 3',
4: 'Exit',
}
def print_menu():
for key in menu_options.keys():
print (key, '--', menu_options[key] )
def option1():
print('Handle option \'Option 1\'')
def option2():
print('Handle option \'Option 2\'')
def option3():
print('Handle option \'Option 3\'')
if __name__=='__main__':
while(True):
print_menu()
option = ''
try:
option = int(input('Enter your choice: '))
except:
print('Wrong input. Please enter a number ...')
#Check what choice was entered and act accordingly
if option == 1:
option1()
elif option == 2:
option2()
elif option == 3:
option3()
elif option == 4:
print('Thanks message before exiting')
exit()
else:
print('Invalid option. Please enter a number between 1 and 4.')
I hope this code is helpful for you.
You can also find the previous code in github.
Practice makes perfection
Create a console application that allow the user to choose from two choices:
1- Print the digits of a number from right to left without using string data type. You can see an explanation of this problem, and the solution, in this link.
2- Print the Pascal Triangle using strings and loops. Find an explanation on how to do it in this link.
H@ppy coding!!
Related posts:
OOP for beginners: A practical example in python
Learning Object-Oriented Programming in Python
Software crash: exception handling in python
H@ppy coding!