In this tutorial, you will learn how to create a multiplication table in python. It is one of the many examples that helps you to practice how to work with loops in any programming language.
See below a picture of what we are going to create in this tutorial.
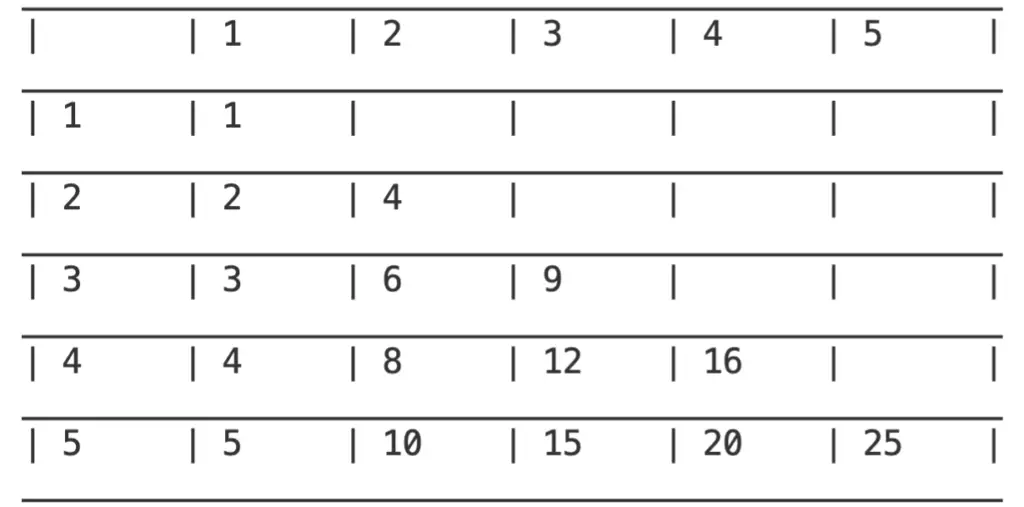
As you can see in the picture above, our multiplication table will have as the first column and first row the number for which we are creating the multiplication table.
Then, the intersection of row and column is the value of row * column. For example, at the intersection of column 2 and row 3, you will find a 6, because 2*3=6.
The empty values are already calculated in another place. For instance, the intersection of column 3 and row 2 is empty because that value is already calculated and shown at the intersection of column 2 and row 3.
Also, notice that we are printing lines to make the table easy to read.
Let’s start our implementation.
Printing horizontal lines
As in my previous post, we are going to apply a Divide and Conquer strategy.
From the example, we can see that the horizontal lines are not bounded to the numerical calculations. So, let’s create a function to print the horizontal lines and we can use it later after printing each row of numbers.
CELL_SIZE = 6
def print_horizontal_line(size):
print('_' * ((size +1) * (CELL_SIZE+2)+1))
For this implementation, we will assume that when we call the function, the cursor is at the beginning of the line that has to be printed.
Python gives us the possibility of printing a string several times just by multiplying the string for the number of times we want. In a programming language without this feature, you can just use a for-loop from 0 to “(size +1) * (CELL_SIZE+2)+1” and then print the character ‘_’.
Print the multiplication table in python
From the figure, we can see the table have the same number of rows and columns. Also, that view gives us a two-dimensional sense of the data we need to print. One dimension is the rows and the other one is the columns.
Every time we have a situation like this, we should know that we have to use nested loops. If we have two dimensions, we use two nested loops. If we have three dimensions, we use three nested loops, and so on.
Let’s start our design.
First, we are going to print the first row. These are the numbers 1, 2, 3 until size. Notice that size is the size of the table.
In our case, we are creating a console application. So, the mode we are using to print is text mode. It won’t look as nice as if we were using graphic mode. The lines won’t be exactly connected to each other.
We will use the character ‘|’ to separate the columns and ‘_’ to separate the rows.
Below is the code to print the first row. Notice that in this case we just print the numbers 1, 2, … size.
We use “,end=spaces” in the function print to keep the cursor on the same line.
print_horizontal_line(size) # first line of the table
print('|',' '*CELL_SIZE, end='')
for col in range(size):
spaces = ' ' * (CELL_SIZE - len(str((col+1))))
print('|',col+1, end=spaces)
print('|')
After we print the first row, we can start calculating the multiplication value of each cell.
print_horizontal_line(size)
for row in range(size):
spaces = ' ' * (CELL_SIZE - len(str((row+1))))
print('|', row+1, end=spaces)
for col in range(size):
spaces = ' ' * (CELL_SIZE - len(str((row + 1) * (col+1))))
if (col<row+1):
print('|',(row + 1) * (col+1), end=spaces)
else:
spaces = ' ' * (CELL_SIZE+1)
print('|', end=spaces)
print('|')
print_horizontal_line(size)
From the code above, notice that there are two nested for-loops. I already explained why we need two nested loops.
For each row (inside of the for-loop), we first print the character ‘|’ so to open the table on that specific row. After that, we print the row number (1, 2, 3, … size) and then we complete the cell with empty spaces.
An interesting thing here is to know how many empty spaces to print, to get all the cells of the same size.
Notice that some cells will have a one-digit number, some two digits and so on, depending on the size of the table.
Let’s assume we decide we want a table that has 6 spaces on each cell. If we print a one-digit number, then we have to print 5 empty spaces. But if we print a two-digit number, then we print only 4 empty spaces and so on.
For that reason, we calculate the spaces of each cell. In the first column, we do not multiply any numbers, but for the rest of the columns, we do it. So, we need two different formulas to calculate the spaces. One for the first column and another one for the rest. This explains the two ways spaces are calculated at the beginning of each loop.
Another thing we have to consider is that we do not repeat calculations. That is, some cells will be empty. In this case, we have to calculate the spaces in another way because we will only print empty spaces.
After the nested for-loop, we have to print the character ‘|’ “to close” that row. And when we close the row then we print a new horizontal line to separate the row from the next one.
All that is left now is the main program.
if __name__ == '__main__':
print_multiplication_table(5)
You can change the size of the table to a bigger or a smaller number.
Feel free to use this code in the way you need.
Find the full code here.
H@ppy coding!
Related posts: